BoxedApp Packer 2018.10.0.0/SDK 3.3.5.22/API 3.3.0.14
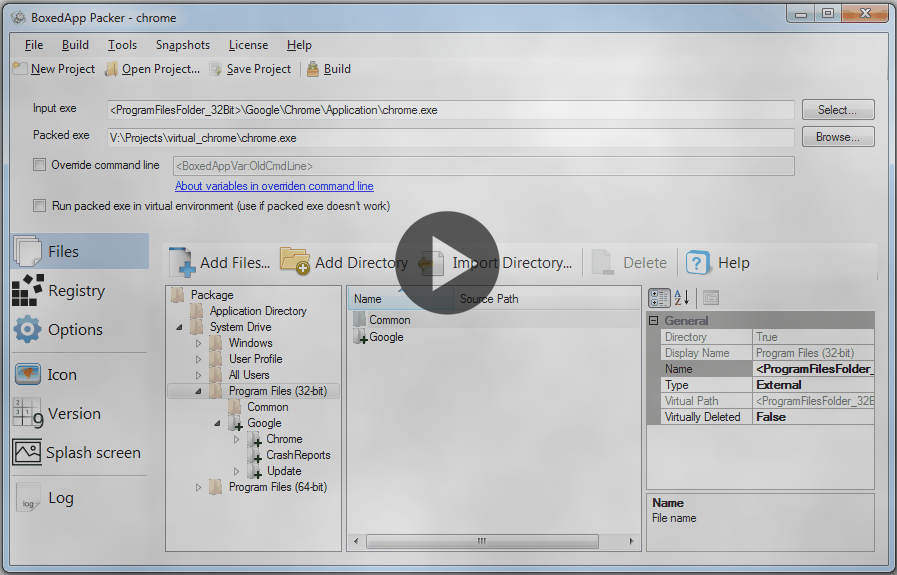
BoxedApp Packer 2018.10.0.0/SDK 3.3.5.22/API 3.3.0.14
BoxedApp is a developer library that provides a set of functions for emulating a file system and a system registry for an application. Using these functions, you can create virtual files, fake registry entries, keys and values. You can launch processes from memory directly, use ActiveX without registration, embed runtimes like .Net, Flash and VC++ redistributable. Supports both x86 and x64 platforms. Samples for C++, Delphi, VB6, C#, VB.Net are available.
Why and when is it useful?
When an application must run properly even if it doesn't have the right to write to the system registry and to the file system.
When an application uses DLL and files, which are to be kept secure, and because of that you can't save them to disk.
When an application needs ActiveX but doesn't have an installer because it must run instantly, without the installation (for example, when it's a portable application that runs from a flash card).
Just a quick example: suppose, your application uses a Flash ActiveX player to display a Flash movie or video. The end users would need a Flash player ActiveX to allow your application work properly. Also, keep in mind that Flash player is not capable of loading files directly from memory. That exposes two major problems: first, you would have to install a Flash player ActiveX, and second, you would have to have the movie in a file. BoxedApp SDK solves these problems: you simply create a virtual file that contains the flash movie, another virtual file that contains the Flash player ActiveX DLL, and virtual registry entries that point to that virtual file. That's it. Now the application "thinks" that that the Flash player ActiveX is actually installed, so the Flash player works just as if the movie file was actually there.
What is BoxedApp SDK?
BoxedApp SDK is a developer library that provides a set of functions for emulating a file system and a system registry for an application. Using these functions, you can create virtual files, fake registry entries, keys and values. You can launch processes from memory directly, use ActiveX without registration, embed runtimes like .Net, Flash and VC++ redistributable.
Supports both x86 and x64 platforms.
Samples for C++, Delphi, VB6, C#, VB.Net are available.
Use Cases
Play Video that Takes Content from Encrypted Source
Why and when is it useful?
When an application must run properly even if it doesn't have the right to write to the system registry and to the file system.
When an application uses DLL and files, which are to be kept secure, and because of that you can't save them to disk.
When an application needs ActiveX but doesn't have an installer because it must run instantly, without the installation (for example, when it's a portable application that runs from a flash card).
Just a quick example: suppose, your application uses a Flash ActiveX player to display a Flash movie or video. The end users would need a Flash player ActiveX to allow your application work properly. Also, keep in mind that Flash player is not capable of loading files directly from memory. That exposes two major problems: first, you would have to install a Flash player ActiveX, and second, you would have to have the movie in a file. BoxedApp SDK solves these problems: you simply create a virtual file that contains the flash movie, another virtual file that contains the Flash player ActiveX DLL, and virtual registry entries that point to that virtual file. That's it. Now the application "thinks" that that the Flash player ActiveX is actually installed, so the Flash player works just as if the movie file was actually there.
In other words, you can now embed all DLL and content files, all ActiveX and OCX components, which your application uses, into a single EXE file. BoxedApp SDK doesn't unpack these files to disk; it doesn't use temporary files either.
Creating Virtual Files
// BoxedAppSDK_CreateVirtualFile creates virtual file
HANDLE hVirtualFile =
BoxedAppSDK_CreateVirtualFile(
_T("C:\\1.swf"),
GENERIC_READ,
FILE_SHARE_READ,
NULL,
CREATE_NEW,
0,
NULL);
// Get pointer to a resource and its size
HMODULE hModule = GetModuleHandle(NULL);
HRSRC hResInfo = FindResource(hModule, _T("BIN1"), _T("BIN"));
HGLOBAL hResData = LoadResource(hModule, hResInfo);
LPVOID lpData = LockResource(hResData);
DWORD dwSize = SizeofResource(hModule, hResInfo);
// Write to just created virtual file
DWORD dwTemp;
WriteFile(hVirtualFile, lpData, dwSize, &dwTemp, NULL);
// Let's close handle
CloseHandle(hVirtualFile);
Creating Virtual Registry Keys
// Create hKey_Classes to work well even under registry reflection
HKEY hKey_Classes;
RegOpenKey(HKEY_LOCAL_MACHINE, _T("SOFTWARE\\Classes"), &hKey_Classes);
HKEY hVirtualKey;
DWORD dwDisposition;
LONG lResult =
BoxedAppSDK_CreateVirtualRegKey(
hKey_Classes,
_T("Typelib\\{D27CDB6B-AE6D-11CF-96B8-444553540000}"),
0,
NULL,
REG_OPTION_NON_VOLATILE,
KEY_ALL_ACCESS,
NULL,
&hVirtualKey,
&dwDisposition
);
HKEY hVirtualKey__10;
lResult =
BoxedAppSDK_CreateVirtualRegKey(
hKey_Classes,
_T("Typelib\\{D27CDB6B-AE6D-11CF-96B8-444553540000}\\1.0"),
0,
NULL,
REG_OPTION_NON_VOLATILE,
KEY_ALL_ACCESS,
NULL,
&hVirtualKey__10,
&dwDisposition
);
TCHAR buf[1024] = _T("Shockwave Flash");
RegSetValueEx(
hVirtualKey__10,
_T(""),
0,
REG_SZ,
(CONST BYTE*)buf,
(lstrlen(buf) + 1) * sizeof(TCHAR));
API for intercepting functions
To create a virtual file system / registry, BoxedApp SDK (this, by the way, is exactly what applications created with BoxedApp Packer use) uses the interception of system functions technique. A number of original ideas have allowed us to create an interception system compatible with any environment, and now the part of SDK that is in charge of the interception has become accessible to developers - SDK users.
typedef HANDLE (WINAPI *P_CreateFileW)(
LPCWSTR lpFileName,
DWORD dwDesiredAccess,
DWORD dwShareMode,
LPSECURITY_ATTRIBUTES lpSecurityAttributes,
DWORD dwCreationDisposition,
DWORD dwFlagsAndAttributes,
HANDLE hTemplateFile);
P_CreateFileW g_pCreateFileW;
HANDLE WINAPI My_CreateFileW(
LPCWSTR lpFileName,
DWORD dwDesiredAccess,
DWORD dwShareMode,
LPSECURITY_ATTRIBUTES lpSecurityAttributes,
DWORD dwCreationDisposition,
DWORD dwFlagsAndAttributes,
HANDLE hTemplateFile)
{
if (0 == lstrcmpiW(lpFileName, L"1.txt"))
{
SetLastError(ERROR_FILE_EXISTS);
return INVALID_HANDLE_VALUE;
}
else
return g_pCreateFileW(
lpFileName,
dwDesiredAccess,
dwShareMode,
lpSecurityAttributes,
dwCreationDisposition,
dwFlagsAndAttributes,
hTemplateFile);
}
...
BoxedAppSDK_Init();
PVOID pCreateFileW = GetProcAddress(GetModuleHandle(_T("kernel32.dll")), "CreateFileW");
HANDLE hHook__CreateFileW = BoxedAppSDK_HookFunction(pCreateFileW, &My_CreateFileW, TRUE);
g_pCreateFileW = (P_CreateFileW)BoxedAppSDK_GetOriginalFunction(hHook__CreateFileW);
FILE* f = fopen("1.txt", "r");
// f is NULL
...
BoxedAppSDK_UnhookFunction(hHook__CreateFileW);
Delphi version of the same idea:
type
TCreateFileW =
function(lpFileName: PWideChar;
dwDesiredAccess, dwShareMode: Integer;
lpSecurityAttributes: PSecurityAttributes;
dwCreationDisposition, dwFlagsAndAttributes: DWORD;
hTemplateFile: THandle): THandle; stdcall;
var
OriginalCreateFileW: TCreateFileW;
function My_CreateFileW(
lpFileName: PWideChar;
dwDesiredAccess, dwShareMode: Integer;
lpSecurityAttributes: PSecurityAttributes;
dwCreationDisposition, dwFlagsAndAttributes: DWORD;
hTemplateFile: THandle): THandle; stdcall;
begin
if 0 = lstrcmpiW(lpFileName, '1.txt') then
begin
Result := INVALID_HANDLE_VALUE;
SetLastError(ERROR_ALREADY_EXISTS);
end
else
Result :=
OriginalCreateFileW(
lpFileName,
dwDesiredAccess,
dwShareMode,
lpSecurityAttributes,
dwCreationDisposition,
dwFlagsAndAttributes,
hTemplateFile);
end;
var
pCreateFileW: Pointer;
hHook__CreateFileW: THandle;
begin
Application.Initialize;
BoxedAppSDK_Init;
pCreateFileW := GetProcAddress(GetModuleHandle('kernel32.dll'), 'CreateFileW');
hHook__CreateFileW := BoxedAppSDK_HookFunction(pCreateFileW, @My_CreateFileW, TRUE);
OriginalCreateFileW := BoxedAppSDK_GetOriginalFunction(hHook__CreateFileW);
// This line produces an exception because we prevent creating / opening '1.txt'
TFileStream.Create('1.txt', fmCreate or fmOpenRead);
BoxedAppSDK_UnhookFunction(hHook__CreateFileW);
Embedding DLL
HANDLE hFile__DLL1 =
BoxedAppSDK_CreateVirtualFile(
_T("Z:\\DLL1.dll"),
GENERIC_WRITE,
FILE_SHARE_READ,
NULL,
CREATE_NEW,
0,
NULL
);
DWORD dwTemp;
WriteFile(hFile__DLL1, pBuffer, dwSize, &dwTemp, NULL);
CloseHandle(hFile__DLL1);
// ...
HMODULE hModule = LoadLibrary(_T("Z:\\DLL1.dll"));
typedef void (WINAPI *P_Function)();
P_Function pFunction = (P_Function)GetProcAddress(hModule, "Function");
pFunction();
FreeLibrary(hModule);


Warning! You are not allowed to view this text.